一、效果如图:
二、界面
<Window x:Class="WpfHelper.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:model="clr-namespace:WpfHelper"
Title="文件模型属性" Height="400" Width="500"
xmlns:lc="http://schemas.devexpress.com/winfx/2008/xaml/layoutcontrol"
xmlns:dxe="http://schemas.devexpress.com/winfx/2008/xaml/editors"
>
<Grid>
<Grid.Resources>
<HierarchicalDataTemplate DataType="{x:Type model:NodeEntry}" ItemsSource="{Binding NodeEntrys}">
<StackPanel Orientation="Horizontal" Margin="0,2,0,2">
<CheckBox Focusable="False"
///说明Mode=TwoWay} 绑定模式,双向绑定
VerticalAlignment="Center" IsChecked="{Binding IsChecked, Mode=TwoWay}"/>
<TextBlock Text="{Binding Name}" ToolTip="{Binding Name}" Tag="{Binding ID}"/>
</StackPanel>
<HierarchicalDataTemplate.ItemContainerStyle>
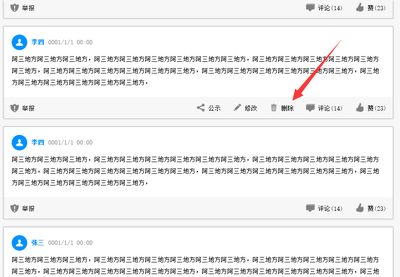
<Style TargetType="TreeViewItem">
<Setter Property="IsExpanded" Value="True" />
</Style>
</HierarchicalDataTemplate.ItemContainerStyle>
</HierarchicalDataTemplate>
</Grid.Resources>
<Grid.ColumnDefinitions>
<ColumnDefinition MinWidth="100" Width="170*"/>
<ColumnDefinition Width="1*" />
<ColumnDefinition Width="80" />
<ColumnDefinition Width="80" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="40*" />
<RowDefinition Height="40" />
</Grid.RowDefinitions>
<GridSplitter Grid.Column="1" Grid.RowSpan="5" Name="gridSplitter1" HorizontalAlignment="Stretch" />
<Button Content="确定" Grid.Column="2" Grid.Row="1" Height="25" HorizontalAlignment="right" Margin="0,10,0,0" Name="btnOK" Click="btnOK_Click" VerticalAlignment="Top" Width="50" IsDefault="True" />
<Button Content="取消" Grid.Column="3" Grid.Row="1" Height="25" HorizontalAlignment="right" Margin="0,10,10,0" Name="btnCancel" Click="btnCancel_Click" VerticalAlignment="Top" Width="50" IsCancel="True" />
<TreeView Grid.ColumnSpan="2" Name="treeView1" MouseRightButtonDown="treeView1_MouseRightButtonDown">
</TreeView>
<TreeView Grid.Column="2" Grid.ColumnSpan="2" Name="treeView2" FocusableChanged="treeView2_FocusableChanged" SelectedItemChanged="treeView2_SelectedItemChanged">
</TreeView>
</Grid>
</Window>
三、绑定的实体类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ComponentModel;
namespace WpfHelper
{
public class NodeEntry : INotifyPropertyChanged
{
public NodeEntry()
{
this.NodeEntrys = new List<NodeEntry>();
this.ParentID = -1;
this.IsChecked = true;
}
int id;
public int ID
{
get { return id; }
set { id = value; this.OnPropertyChanged("ID"); }
}
string name;
public string Name
{
get { return name; }
set { name = value; this.OnPropertyChanged("Name"); }
}
public int ParentID { get; set; }
bool isChecked;
public bool IsChecked
{
get { return isChecked; }
set { isChecked = value; this.OnPropertyChanged("IsChecked"); }
}
List<NodeEntry> nodeEntrys;
public List<NodeEntry> NodeEntrys
{
get { return nodeEntrys; }
set { nodeEntrys = value;
this.OnPropertyChanged("NodeEntrys");
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(string prop)
{
if (this.PropertyChanged != null)
this.PropertyChanged(this, new PropertyChangedEventArgs(prop));
// System.Windows.MessageBox.Show(prop);
}
}
}
四、窗体实现
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Data;
using DevExpress.Xpf.Editors;
namespace WpfHelper
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
List<NodeEntry> m_NodeEntrys;
List<NodeEntry> m_outputList;
public MainWindow()
{
InitializeComponent();
m_NodeEntrys = new List<NodeEntry>()
{
new NodeEntry { ID = 2, Name = "北京市", ParentID = 1 },
new NodeEntry { ID = 1, Name = "中国" },
new NodeEntry { ID = 3, Name = "吉林省", ParentID = 1 },
new NodeEntry { ID = 4, Name = "上海市", ParentID = 1 },
new NodeEntry { ID = 5, Name = "海淀区", ParentID = 2 },
new NodeEntry { ID = 6, Name = "朝阳区", ParentID = 2 },
new NodeEntry { ID = 7, Name = "大兴区", ParentID = 2 },
new NodeEntry { ID = 8, Name = "白山市", ParentID = 3 },
new NodeEntry { ID = 9, Name = "长春市", ParentID = 3 },
new NodeEntry { ID = 10, Name = "抚松县", ParentID = 8 },
new NodeEntry { ID = 11, Name = "靖宇县", ParentID = 8 },
new NodeEntry { ID = 13, Name = "靖宇县" }
};
m_outputList = Bind(m_NodeEntrys);
this.treeView1.ItemsSource = m_outputList;
this.treeView2.ItemsSource = m_outputList;
}
List<NodeEntry> Bind(List<NodeEntry> nodes)
{
List<NodeEntry> outputList = new List<NodeEntry>();
for (int i = 0; i < nodes.Count; i++)
{
nodes[i].IsChecked = false;
if (nodes[i].ParentID == -1) outputList.Add(nodes[i]);
else FindDownward(nodes, nodes[i].ParentID).NodeEntrys.Add(nodes[i]);
}
return outputList;
}
NodeEntry FindDownward(List<NodeEntry> nodes, int id)
{
if (nodes == null) return null;
for (int i = 0; i < nodes.Count; i++)
{
if (nodes[i].ID == id)
{
return nodes[i];
}
//NodeEntry node = FindDownward(nodes[i].NodeEntrys, id);
//if (node != null) return node;
}
return null;
}
private void btnOK_Click(object sender, RoutedEventArgs e)
{
try
{
m_NodeEntrys.Add(new NodeEntry { ID = 14, IsChecked = true, Name = "法国" });
m_outputList.Add(new NodeEntry { ID = 14, IsChecked = true, Name = "法国" });
//m_outputList = Bind(m_NodeEntrys);
NodeEntry node = new NodeEntry();
this.treeView1.ItemsSource = m_outputList;
this.treeView2.ItemsSource = null;
this.treeView2.ItemsSource = m_outputList;
}
catch(Exception ex)
{
}
}
private void btnCancel_Click(object sender, RoutedEventArgs e)
{
}
//双向绑定改名,选择
private void treeView2_SelectedItemChanged(object sender, RoutedPropertyChangedEventArgs<object> e)
{
NodeEntry item = (NodeEntry)this.treeView2.SelectedItem;
item.Name = "dido";
item.IsChecked = true;
MessageBox.Show(item.ID.ToString());
}
}
}