Searches for two adjacent elements that are equal.
binary_search (STL/CLR)
Tests whether a sorted sequence contains a given value.
copy (STL/CLR)
Copies values from a source range to a destination range, iteratingin the forward direction.
copy_backward (STL/CLR)
Copies values from a source range to a destination range, iteratingin the backward direction.
count (STL/CLR)
Returns the number of elements in a range whose values match aspecified value.
count_if (STL/CLR)
Returns the number of elements in a range whose values match aspecified condition.
equal (STL/CLR)
Compares two ranges, element by element.
equal_range (STL/CLR)
Searches an ordered sequence of values and returns two positionsthat delimit a subsequence of values that are all equal to a givenelement.
fill (STL/CLR)
Assigns the same new value to every element in a specifiedrange.
fill_n (STL/CLR)
Assigns a new value to a specified number of elements in a rangebeginning with a particular element.
find (STL/CLR)
Returns the position of the first occurrence of a specifiedvalue.
find_end (STL/CLR)
Returns the last subsequence in a range that is identical to aspecified sequence.
find_first_of (STL/CLR)
Searches a range for the first occurrence of any one of a givenrange of elements.
find_if (STL/CLR)
Returns the position of the first element in a sequence of valueswhere the element satisfies a specified condition.
for_each (STL/CLR)
Applies a specified function object to each element in a sequenceof values and returns the function object.
generate (STL/CLR)
Assigns the values generated by a function object to each elementin a sequence of values.
generate_n (STL/CLR)
Assigns the values generated by a function object to a specifiednumber of elements.
includes (STL/CLR)
Tests whether one sorted range contains all the elements in asecond sorted range.
inplace_merge (STL/CLR)
Combines the elements from two consecutive sorted ranges into asingle sorted range.
iter_swap (STL/CLR)
Exchanges two values referred to by a pair of specifiediterators.
lexicographical_compare (STL/CLR)
Compares two sequences, element by element, identifying whichsequence is the lesser of the two.
lower_bound (STL/CLR)
Finds the position of the first element in an ordered sequence ofvalues that has a value greater than or equal to a specifiedvalue.
make_heap (STL/CLR)
Converts elements from a specified range into a heap where thefirst element on the heap is the largest.
max (STL/CLR)
Compares two objects and returns the greater of the two.
max_element (STL/CLR)
Finds the largest element in a specified sequence of values.
merge (STL/CLR)
Combines all the elements from two sorted source ranges into asingle, sorted destination range.
min (STL/CLR)
Compares two objects and returns the lesser of the two.
min_element (STL/CLR)
Finds the smallest element in a specified sequence of values.
mismatch (STL/CLR)
Compares two ranges element by element and returns the firstposition where a difference occurs.
next_permutation (STL/CLR)
Reorders the elements in a range so that the original ordering isreplaced by the lexicographically next greater permutation if itexists.
nth_element (STL/CLR)
Partitions a sequence of elements, correctly locating the nthelement of the sequence so that all the elements in front of it areless than or equal to it and all the elements that follow it aregreater than or equal to it.
partial_sort (STL/CLR)
Arranges a specified number of the smaller elements in a range intonondescending order.
partial_sort_copy (STL/CLR)
Copies elements from a source range into a destination range suchthat the elements from the source range are ordered.
partition (STL/CLR)
Arranges elements in a range such that those elements that satisfya unary predicate precede those that fail to satisfy it.
pop_heap (STL/CLR)
Moves the largest element from the front of a heap to the end andthen forms a new heap from the remaining elements.
prev_permutation (STL/CLR)
Reorders a sequence of elements so that the original ordering isreplaced by the lexicographically previous greater permutation ifit exists.
push_heap (STL/CLR)
Adds an element that is at the end of a range to an existing heapconsisting of the prior elements in the range.
random_shuffle (STL/CLR)
Rearranges a sequence of N elements in a range into one of N!possible arrangements selected at random.
remove (STL/CLR)
Deletes a specified value from a given range without disturbing theorder of the remaining elements and returns the end of a new rangefree of the specified value.
remove_copy (STL/CLR)
Copies elements from a source range to a destination range, exceptthat elements of a specified value are not copied, withoutdisturbing the order of the remaining elements.
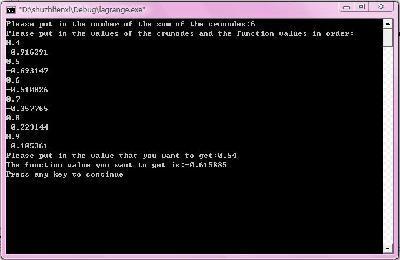
remove_copy_if (STL/CLR)
Copies elements from a source range to a destination range, exceptthose that satisfy a predicate, without disturbing the order of theremaining elements.
remove_if (STL/CLR)
Deletes elements that satisfy a predicate from a given rangewithout disturbing the order of the remaining elements. .
replace (STL/CLR)
Replaces elements in a range that match a specified value with anew value.
replace_copy (STL/CLR)
Copies elements from a source range to a destination range,replacing elements that match a specified value with a newvalue.
replace_copy_if (STL/CLR)
Examines each element in a source range and replaces it if itsatisfies a specified predicate while copying the result into a newdestination range.
replace_if (STL/CLR)
Examines each element in a range and replaces it if it satisfies aspecified predicate.
reverse (STL/CLR)
Reverses the order of the elements within a range.
reverse_copy (STL/CLR)
Reverses the order of the elements within a source range whilecopying them into a destination range.
rotate (STL/CLR)
Exchanges the elements in two adjacent ranges.
rotate_copy (STL/CLR)
Exchanges the elements in two adjacent ranges within a source rangeand copies the result to a destination range.
search (STL/CLR)
Searches for the first occurrence of a sequence within a targetrange whose elements are equal to those in a given sequence ofelements or whose elements are equivalent in a sense specified by abinary predicate to the elements in the given sequence.
search_n (STL/CLR)
Searches for the first subsequence in a range that of a specifiednumber of elements having a particular value or a relation to thatvalue as specified by a binary predicate.
set_difference (STL/CLR)
Unites all of the elements that belong to one sorted source range,but not to a second sorted source range, into a single, sorteddestination range, where the ordering criterion may be specified bya binary predicate.
set_intersection (STL/CLR)
Unites all of the elements that belong to both sorted source rangesinto a single, sorted destination range, where the orderingcriterion may be specified by a binary predicate.
set_symmetric_difference (STL/CLR)
Unites all of the elements that belong to one, but not both, of thesorted source ranges into a single, sorted destination range, wherethe ordering criterion may be specified by a binarypredicate.
set_union (STL/CLR)
Unites all of the elements that belong to at least one of twosorted source ranges into a single, sorted destination range, wherethe ordering criterion may be specified by a binarypredicate.
sort (STL/CLR)
Arranges the elements in a specified range into a nondescendingorder or according to an ordering criterion specified by a binarypredicate.
sort_heap (STL/CLR)
Converts a heap into a sorted range.
stable_partition (STL/CLR)
Classifies elements in a range into two disjoint sets, with thoseelements satisfying a unary predicate preceding those that fail tosatisfy it, preserving the relative order of equivalentelements.
stable_sort (STL/CLR)
Arranges the elements in a specified range into a nondescendingorder or according to an ordering criterion specified by a binarypredicate and preserves the relative ordering of equivalentelements.
swap (STL/CLR)
Exchanges the values of the elements between two types of objects,assigning the contents of the first object to the second object andthe contents of the second to the first.
swap_ranges (STL/CLR)
Exchanges the elements of one range with the elements of another,equal sized range.
transform (STL/CLR)
Applies a specified function object to each element in a sourcerange or to a pair of elements from two source ranges and copiesthe return values of the function object into a destinationrange.
unique (STL/CLR)
Removes duplicate elements that are adjacent to each other in aspecified range.
unique_copy (STL/CLR)
Copies elements from a source range into a destination range exceptfor the duplicate elements that are adjacent to each other.
upper_bound (STL/CLR)
Finds the position of the first element in an ordered range thathas a value that is greater than a specified value, where theordering criterion may be specified by a binary predicate.