★
C#实现百叶窗效果显示图片
百叶窗有两种显示效果,一种是垂直百叶窗,另一种是水平百叶窗。
实现百叶窗显示图像有两种方式:一是根据窗口或图像的高度或宽度和定制的百叶窗显示条宽度计算百叶窗的显示的条数量;二是根据窗口或图像的高度或宽度和定制的百叶窗显示条数量计算百叶窗的显示的条宽度。
垂直百叶窗实现代码如下:
private void button1_Click(object sender, EventArgs e)
{
//垂直百叶窗显示图像
try
{
MyBitmap = (Bitmap)this.pictureBox1.Image.Clone();
int dw = MyBitmap.Width / 30;
int dh = MyBitmap.Height;
Graphics g = this.pictureBox1.CreateGraphics();
g.Clear(Color.Gray);
Point[] MyPoint = new Point[30];
for (int x = 0; x < 30; x++)
{
MyPoint[x].Y = 0;
MyPoint[x].X = x * dw;
}
Bitmap bitmap = new Bitmap(MyBitmap.Width, MyBitmap.Height);
for (int i = 0; i < dw; i++)
{
for (int j = 0; j < 30; j++)
{
for (int k = 0; k < dh; k++)
{
bitmap.SetPixel(MyPoint[j].X + i, MyPoint[j].Y
MyBitmap.GetPixel(MyPoint[j].X + i, MyPoint[j].Y + k));
} + k,
}
this.pictureBox1.Refresh();
this.pictureBox1.Image = bitmap;
System.Threading.Thread.Sleep(100);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示");
}
}
水平百叶窗实现代码如下:
private void button3_Click(object sender, EventArgs e)
{
//水平百叶窗显示图像
try
{
MyBitmap = (Bitmap)this.pictureBox1.Image.Clone();
int dh = MyBitmap.Height / 20;
int dw = MyBitmap.Width;
Graphics g = this.pictureBox1.CreateGraphics();
g.Clear(Color.Gray);
Point[] MyPoint = new Point[20];
for (int y = 0; y < 20; y++)
{
MyPoint[y].X = 0;
MyPoint[y].Y = y * dh;
}
Bitmap bitmap = new Bitmap(MyBitmap.Width, MyBitmap.Height); for (int i = 0; i < dh; i++)
{
for (int j = 0; j < 20; j++)
{
for (int k = 0; k < dw; k++)
{
bitmap.SetPixel(MyPoint[j].X + k, MyPoint[j].Y MyBitmap.GetPixel(MyPoint[j].X + k, MyPoint[j].Y + i));
}
}
this.pictureBox1.Refresh(); + i,
this.pictureBox1.Image = bitmap;
System.Threading.Thread.Sleep(100);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示");
}
}
★
C#实现淡入淡出显示图像效果显示图片
以淡入淡出显示图像效果显示图像主要使用了ImageAttributes类的SetColorMatrix方法设置颜色调整矩阵实现淡入淡出的效果。ImageAttributes对象维护多个颜色调整设置,包括颜色调整矩阵、灰度调整矩阵、灰度校正值、颜色映射表和颜色阈值。呈现过程中,可以对颜色进行校正、调暗、调亮和移除。
淡入效果代码如下:
private void button1_Click(object sender, EventArgs e)
{
//淡入显示图像
try
{
Graphics g = this.panel1.CreateGraphics();
g.Clear(Color.Gray);
int width = MyBitmap.Width;
int height = MyBitmap.Height;
ImageAttributes attributes = new ImageAttributes();
ColorMatrix matrix = new ColorMatrix();
//创建淡入颜色矩阵
matrix.Matrix00 = (float)0.0;
matrix.Matrix01 = (float)0.0;
matrix.Matrix02 = (float)0.0;
matrix.Matrix03 = (float)0.0;
matrix.Matrix04 = (float)0.0;
matrix.Matrix10 = (float)0.0;
matrix.Matrix11 = (float)0.0;
matrix.Matrix12 = (float)0.0;
matrix.Matrix13 = (float)0.0;
matrix.Matrix14 = (float)0.0;
matrix.Matrix20 = (float)0.0;
matrix.Matrix21 = (float)0.0;
matrix.Matrix22 = (float)0.0;
matrix.Matrix23 = (float)0.0;
matrix.Matrix24 = (float)0.0;
matrix.Matrix30 = (float)0.0;
matrix.Matrix31 = (float)0.0;
matrix.Matrix32 = (float)0.0;
matrix.Matrix33 = (float)0.0;
matrix.Matrix34 = (float)0.0;
matrix.Matrix40 = (float)0.0;
matrix.Matrix41 = (float)0.0;
matrix.Matrix42 = (float)0.0;
matrix.Matrix43 = (float)0.0;
matrix.Matrix44 = (float)0.0;
matrix.Matrix33 = (float)1.0;
matrix.Matrix44 = (float)1.0;
//从0到1进行修改色彩变换矩阵主对角线上的数值
//使三种基准色的饱和度渐增
Single count = (float)0.0;
while (count < 1.0)
{
matrix.Matrix00 = count;
matrix.Matrix11 = count;
matrix.Matrix22 = count;
matrix.Matrix33 = count;
attributes.SetColorMatrix(matrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap);
g.DrawImage(MyBitmap, new Rectangle(0, 0, width, height), 0, 0, width, height, GraphicsUnit.Pixel, attributes);
System.Threading.Thread.Sleep(200);
count = (float)(count + 0.02);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示");
}
}
淡出效果代码如下:
private void button3_Click(object sender, EventArgs e)
{
//淡出显示图像
try
{
Graphics g = this.panel1.CreateGraphics();
g.Clear(Color.Gray);
int width = MyBitmap.Width;
int height = MyBitmap.Height;
ImageAttributes attributes = new ImageAttributes(); ColorMatrix matrix = new ColorMatrix();
//创建淡出颜色矩阵
matrix.Matrix00 = (float)0.0;
matrix.Matrix01 = (float)0.0;
matrix.Matrix02 = (float)0.0;
matrix.Matrix03 = (float)0.0;
matrix.Matrix04 = (float)0.0;
matrix.Matrix10 = (float)0.0;
matrix.Matrix11 = (float)0.0;
matrix.Matrix12 = (float)0.0;
matrix.Matrix13 = (float)0.0;
matrix.Matrix14 = (float)0.0;
matrix.Matrix20 = (float)0.0;
matrix.Matrix21 = (float)0.0;
matrix.Matrix22 = (float)0.0;
matrix.Matrix23 = (float)0.0;
matrix.Matrix24 = (float)0.0;
matrix.Matrix30 = (float)0.0;
matrix.Matrix31 = (float)0.0;
matrix.Matrix32 = (float)0.0;
matrix.Matrix33 = (float)0.0;
matrix.Matrix34 = (float)0.0;
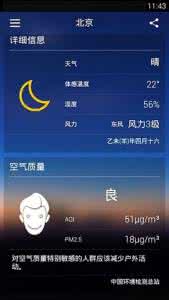
matrix.Matrix40 = (float)0.0;
matrix.Matrix41 = (float)0.0;
matrix.Matrix42 = (float)0.0;
matrix.Matrix43 = (float)0.0;
matrix.Matrix44 = (float)0.0;
matrix.Matrix33 = (float)1.0;
matrix.Matrix44 = (float)1.0;
//从1到0进行修改色彩变换矩阵主对角线上的数值 //依次减少每种色彩分量
Single count = (float)1.0;
while (count > 0.0)
{
matrix.Matrix00 = (float)count;
matrix.Matrix11 = (float)count;
matrix.Matrix22 = (float)count;
matrix.Matrix33 = (float)count;
attributes.SetColorMatrix(matrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap);
g.DrawImage(MyBitmap, new Rectangle(0, 0, width, height), 0, 0, width, height, GraphicsUnit.Pixel, attributes);
System.Threading.Thread.Sleep(20);
count = (float)(count - 0.01);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示");
}
}
★
C#实现任意角度旋转图片
以任意角度旋转图像示例。
实现任意角度旋转图像主要使用Graphics类提供的RotateTransform()方法。代码如下:
private void button1_Click(object sender, EventArgs e)
{
//以任意角度旋转显示图像
Graphics g = this.panel1.CreateGraphics();
float MyAngle = 0;//旋转的角度
while (MyAngle < 360)
{
TextureBrush MyBrush = new TextureBrush(MyBitmap);
this.panel1.Refresh();
MyBrush.RotateTransform(MyAngle);
g.FillRectangle(MyBrush, 0, 0, this.ClientRectangle.Width, this.ClientRectangle.Height); MyAngle += 0.5f;
System.Threading.Thread.Sleep(50);
}
}
★
C#实现浮雕效果显示图片
使图像产生浮雕的效果,主要通过对图像像素点的像素值分别与相邻像素点的像素值相减后加上128,然后将其作为新的像素点的值。
以浮雕效果显示图像主要通过GetPixel方法获得每一点像素的值,通过SetPixel设置该像素点的像素值。代码如下:
private void button1_Click(object sender, EventArgs e)
{
//以浮雕效果显示图像
try
{
int Height = this.pictureBox1.Image.Height;
int Width = this.pictureBox1.Image.Width;
Bitmap newBitmap = new Bitmap(Width, Height);
Bitmap oldBitmap = (Bitmap)this.pictureBox1.Image;
Color pixel1, pixel2;
for (int x = 0; x < Width - 1; x++)
{
for (int y = 0; y < Height - 1; y++)
{
int r = 0, g = 0, b = 0;
pixel1 = oldBitmap.GetPixel(x, y);
pixel2 = oldBitmap.GetPixel(x + 1, y + 1);
r = Math.Abs(pixel1.R - pixel2.R + 128);
g = Math.Abs(pixel1.G - pixel2.G + 128);
b = Math.Abs(pixel1.B - pixel2.B + 128);
if (r > 255)
r = 255;
if (r < 0)
r = 0;
if (g > 255)
g = 255;
if (g < 0)
g = 0;
if (b > 255)
b = 255;
if (b < 0)
b = 0;
newBitmap.SetPixel(x, y, Color.FromArgb(r, g, b));
}
}
this.pictureBox1.Image = newBitmap;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}